What is browser geolocation? How does it relate to geocoding?
Browser geolocation
is a technology supported by almost all web browsers
which enables users to share their location - in the form of geographic
coordinates (longitude and latitude) - with a web service.
Geocoding,
on the other hand, is the process of turning coordinates into geographic
information (address, placenames, etc) or vice versa.
The accuracy of the coordinates obtained from browser geolocation will
depend on the underlying technology
being used to determine the user's location, which could be GPS, WiFi
network, phone cell tower mapping, or IP address. If the user is on a
mobile device with a GPS signal, the coordinates can be highly accurate.
If however the user is using an ISP and the location is coming from an IP
to location lookup the location may not be accurate at all. The browser
location may also be misleading if the user is accessing a web service via
a VPN or similar technologies.
Once you have used browser geolocation to obtain the user's location in
the form of coordinates, these coordinates can be reverse geocoded using
the OpenCage geocoding API to be turned into geographic information like an
address or a country, state/province, time zone, etc.
Please see the code example below or the
OpenCage API reference
for details of how to do the geocoding.
Privacy considerations
Because a user's location is private information, browser geolocation is
only possible if the user explicitly grants permission to a web service
via a browser prompt. You can see an example of what this looks like on
these screenshots.
First, the user is shown a button to start the browser geolocation process:
Then the browser (Firefox in this screenshot) prompts to ask the user if
location info should be shared. Only if the user allows it does the browser
get access to the user's location information.
It is not possible to perform browser geolocation without the user's
active consent.
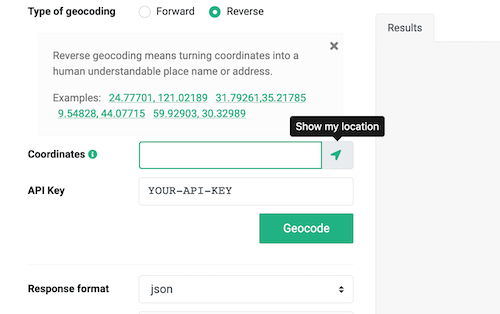
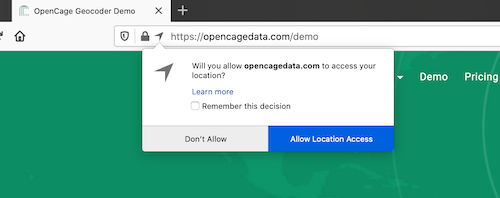
Technical details / Code Example
Most modern web browsers support browser geolocation, but only in a secure
context (HTTPS). Some older browsers may not support browser geolocation.
It is advised to always check in your code whether browser geolocation is
supported before attempting to use the functionality, as shown in this
javascript example.
// browser location starts when this function is called
function getLocation() {
// check to make sure geolocation is possible
if (navigator.geolocation) {
var options = {
enableHighAccuracy: true,
timeout: 5000,
maximumAge: 0
};
navigator.geolocation.getCurrentPosition(success, error, options);
} else {
console.log('Geolocation is not supported'; }
}
}
function error(err) {
console.warn(`ERROR(${err.code}): ${err.message}`);
}
function success(pos) {
var query = pos.coords.latitude + ',' + pos.coords.longitude;
console.log('coordinates: ' + query);
console.log('accuracy: ' + pos.coords.accuracy + ' meters.');
// now we have coordinates, it is time to use them to
// do some reverse geocoding to get back the location information
var api_url = 'https://api.opencagedata.com/geocode/v1/json'
var apikey = 'YOUR-API-KEY';
var request_url = api_url
+ '?'
+ 'key=' + apikey
+ '&q=' + encodeURIComponent(query)
+ '&pretty=1'
+ '&no_annotations=1';
// now we follow the steps in the OpenCage javascript tutorial
// full example:
// https://opencagedata.com/tutorials/geocode-in-javascript
var request = new XMLHttpRequest();
request.open('GET', request_url, true);
request.onload = function() {
// see full list of possible response codes:
// https://opencagedata.com/api#codes
if (request.status === 200){ // Success!
var data = JSON.parse(request.responseText);
alert(data.results[0].formatted);
} else if (request.status <= 500){
// We reached our target server, but it returned an error
console.log("unable to geocode! Response code: " + request.status);
var data = JSON.parse(request.responseText);
console.log('error msg: ' + data.status.message);
} else {
console.log("server error");
}
};
request.onerror = function() {
// There was a connection error of some sort
console.log("unable to connect to server");
};
request.send(); // make the request
}
// trigger getLocation when the page is ready
// change to be when a certain button is clicked or action is taken
$(document).ready(function(){
getLocation();
}
Further Reading
Happy geocoding!
2,500 geocoding API requests/day - No credit card required